In a previous post, I mentioned that I’ve taken 6 weeks off from Lambda in order to improve my JavaScript and React skills. To that end, I grabbed Brad Traversy‘s Modern JavaScript From the Beginning and his React Front to Back courses on Udemy. Yesterday morning, I completed the 2nd unit of the JavaScript course, which dealt with JavaScript Fundamentals. Here’s how it went, and a comparison with what I learned at Lambda so far.
Content
Brad’s course consists of the following units:
Intro & Getting Started covered setting up Visual Studio Code and some extensions, course-specific resources available on GitHub and a general overview of how the course works. It was similar to material from Lambda’s first week of class. Lambda’s coverage was much more in-depth, because we spent more time on learning how to use GitHub in a basic manner which would allow us to clone projects from the site, create our own branches, upload changes, make pull requests, add collaborators, merge to master and so on. I’m not sure yet if Modern JS will delve deeper into GitHub.
With regard to VS Code, there were some differences. I don’t remember Lambda officially going over extensions, but in our individual groups, our TLs did that with us and recommended extensions that we could install. Students and other TLs also shared additional extensions in Slack. Modern JS did go over some shortcuts, which I don’t remember doing at Lambda, but I do remember seeing before in other YouTube videos. I’ll see about making a short post with some shortcuts that I use later.
JavaScript Language Fundamentals
This unit consisted of the following topics. Each had their own video, and a downloadable ZIP file that included HTML and JS code.
- Section Intro & File Setup
- Using The Console
- Variables – var, let & const
- Data Types in JavaScript
- Type Conversion
- Numbers & The Math Object
- String Methods & Concatenation
- Template Literals
- Arrays & Array Methods
- Object Literals
- Dates & Times
- If Statements & Comparison Operators
- Switches
- Function Declarations & Expressions
- General Loops
- A Look At The Window Object
- Block Scope With let & const
My initial impression of the content, after going through it once, is that its better-digested after learning some of this elsewhere – maybe even on Brad’s YouTube channel. Having gone through Lambda’s pre-course and then JavaScript Fundamentals (sprint 3) and Applied JavaScript (sprint 5), much of what I saw in this unit wasn’t new to me, and I was able to follow along with no issues. I do think that people completely new to JavaScript will be a little overwhelmed, because although the topics are all examined on a high-level, there are no exercises for these lessons. The videos are purely informational and actually could have been a general YouTube video refresher series.
With Lambda, we were required to complete and submit exercises and projects that directly utilized the JavaScript topics that we learned every day. I know that projects will come later on in Modern JS, but going through essentially 3.25 hours of videos without doing any coding doesn’t drill these fundamental concepts into our heads the same way. I think that some basic exercises should be included for each of these sections, if its revised in the future. That said, there were some topics covered in this unit that weren’t covered at this stage at Lambda, and some topics also included details that weren’t part of the coursework at Lambda. I’ll delve into these in the given topic’s section, below.
Section Intro & File Setup
This opening video introduces us to JavaScript by listing some of the topics that the section will cover and goes over setting up the coding environment. With VS Code installed, all that the sandbox environment requires is an index.html file and a JavaScript file. It also shows how to include script files, both internally and externally in the HTML file.
One of the shortcuts used in VS Code is typing “!” and hitting the “tab” key to get a boilerplate HTML file. This is only available if Emmet is installed in VS Code, which I think is the case, by default. We’re also shown how to run Live Server, so that changes to our code are automatically made in the browser’s output when changes are saved in VS Code.
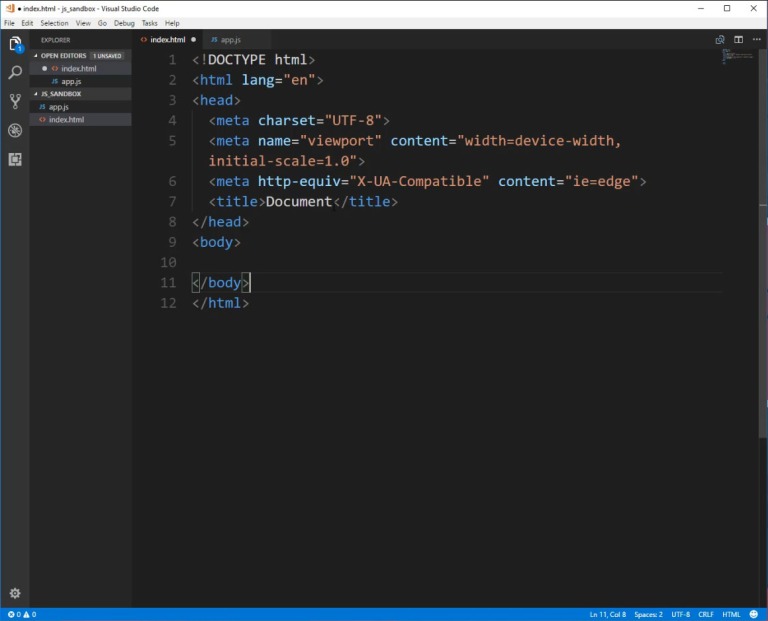
Using The Console
The developer console is a tool built into Chrome that contains information about a given web page. It has multiple tabs, which each house different scopes of information. One of these tabs is the Console, which is a browser-based command line tool. It shows the output of code, like JavaScript, on a web page and can even be used to write JavaScript code, although it can’t save, so once a page is refreshed, any changes made in the console are lost. Because of this, its primarily used for testing and debugging.
Both Modern JS and Lambda’s full-stack precourse show how to open the console in Chrome with the F12 key. I don’t remember either giving a deep explanation about what the console is, though. In Applied JavaScript, at Lambda, when we learned about DOM manipulation, we did look at the console more in-depth, including examining the Document.
Modern JS gave some examples of writing and immediately executing JavaScript in the console, but this included more advanced commands, like query selectors and changing the color of an h1 on the screen via DOM manipulation. Some of this jumping around could be intimidating to new students who have little or no experience coding. I know that if I had seen some of the DOM manipulation when first starting, I would have been confused.
Interestingly, although both the Lambda pre-course and Modern JS have us console.log in the Chrome browser, neither one explains what console.log is. We see its output, in either case, but its not actually explained to us what it means, initially. Modern JS does console.log a variety of different types of data in this lesson, including a string, number, boolean, variable, array and object.
Here are some additional topics discussed in Modern JS:
- single-line comments
- multi-line comments
- changing console text size with control-mousewheel
- console.table()
- console.error()
- console.clear()
- console.warn()
- console.time()
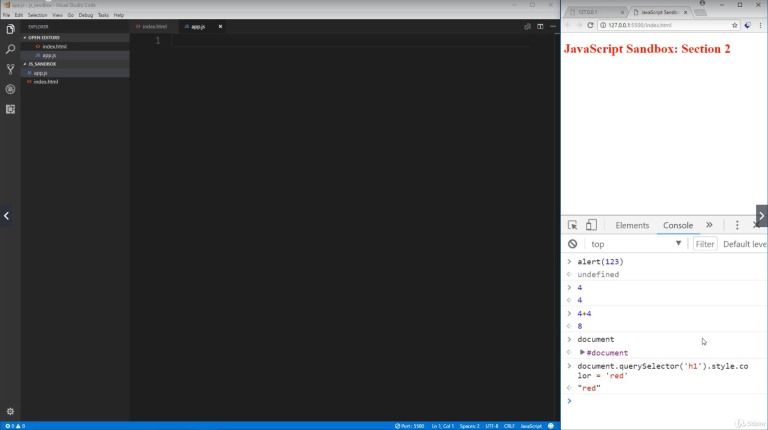
Variables – var, let & const
This video introduces variables in JavaScript and mentions block-level scope, but then defers scope to the last video in this section, because functions and code blocks haven’t yet been covered. It shows how to declare a variable and how to console.log the value of that variable, then reassigns the value of the variable, showing how the newer value overwrites the old one. The concept of a string data type is introduced, but also deferred to the next section, which covers data types in detail.
We see how to initialize a variable (create one and not give it a value) and how that gives it a default value of “undefined” until its assigned a new value. We’re then told that one of the reasons that variables are sometimes initialized but not given an initial value is to support conditional statements (like an if/then statement) which executes different code blocks based on the value of a variable.
Variable names can include letters, numbers, underscores and dollar signs. They can’t begin with a number – doing so results in an error in the console. I don’t remember Lambda mentioning underscores and dollar signs, so that was something new. I think the rest was covered in the pre-course. Modern JS cautions against starting a variable name with a dollar sign or an underscore. Beginning with a dollar sign is something usually reserved for JQuery and and starting with an underscore is generally used for private variables, a concept I’m not yet familiar with.
Some naming conventions are covered, like camel case, Pascal case (which I’ve heard called Snake case, as well), underscore and all lowercase. Lambda mentioned some of these as well, and both recommend camel case, with Pascal case being used for certain things, like constructor functions – which is a common convention among JavaScript coders.
We’re shown how var and let operate similarly when it comes to reassigning values to a variable, and how const is not only fixed, but must be assigned when a const is declared. We then see how values in an object literal that are assigned to a const can be changed and introduced to the idea that if a const is set to reference an object, that can’t be changed, but the data inside of the object can change (or mutate). The const remains unchanged (its still pointed to the same object) but the actual values of data inside of the given object can change. This will go over the heads of absolute beginners, as objects are then deferred to a later video. I remember Lambda going deep into the value of different variables and constants, especially when set to objects and arrays, later in the course – possibly during Advanced State Management, which is the 4th sprint focused on React.
Both Lambda and Modern JS recommend declaring all variables as const, unless they need to change, then use let.
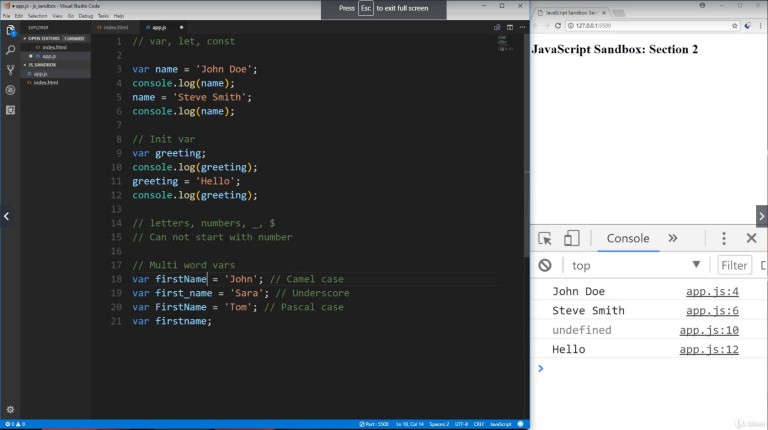
Data Types in JavaScript
This video looks at the types of data that are stored in variables. It goes over primitive data types, which is data that is accessed directly by its value, and reference data types, which is data that is not stored directly where its accessed, but is essentially pointed to. I don’t know the technical details of each of these yet, but the types associated with each are:
Primitive: String, Number, Boolean, Null, Undefined and Symbols (ES6)
Reference: Arrays, Object literals, Functions, Dates
JavaScript is a “dynamically typed” language. This doesn’t mean that we type commands dynamically – its about data types. It means that the “type” of variable is based on the data assigned to it, not the other way around. Other programming languages have developers specify a variable type when the variable is created, like declaring a variable named num and immediately setting it to hold only integer values. JavaScript doesn’t set a type to a variable until data is put into it. For example, we could theoretically have a variable named num and then place a string like “Hello” in it. Its now a string variable.
But that’s not all. We could later assign a value of 30 to num, and it would overwrite the “Hello” value with the value of 30 and change the type from string to number. This is something that really bewildered me when I first learned it in the Lambda pre-course, and then again in the Modern JS course.
The opposite of this, statically-typed languages, is what I was familiar with in high school, back in the 1990s. In Pascal, for example, an integer variable could only ever hold an integer value. It couldn’t even hold a different number type, like a decimal value.
Additional topics discussed:
- typeof (shows the data type of a variable)
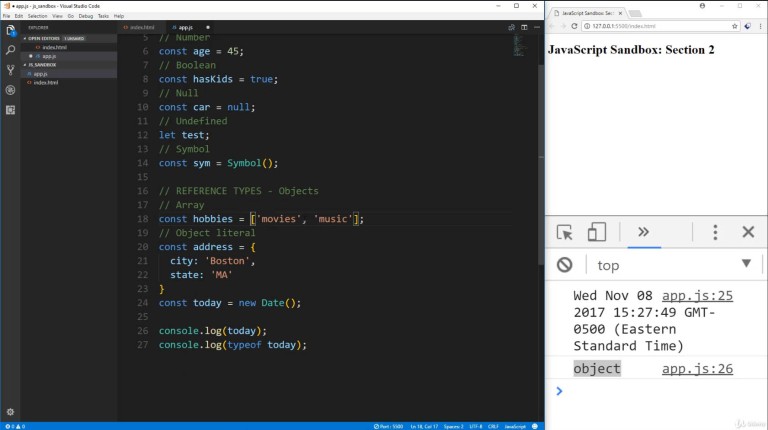
Type Conversion
Type conversion is the process of taking data of one type and converting it into another. What I’ve seen mainly consists of turning numbers into strings, and vice versa. To turn a number into a string, the number is simply wrapped in the String() function. So, for example, to turn the number 213 into a string, we could use the String(213) command. If we then check it with typeof(), we’ll see that its type is no longer “number” but has been converted to “string”. Its .length will also change from “undefined”, which is the .length for all numbers since .length is a string method, to 3, because 213 has 3 characters.
We also get a quick example of type coercion, which is similar to type conversion. Both change the type of a variable to another type. The difference is that coercion is implicit and conversion can be either implicit or explicit. This isn’t explained in the video, but in this case Mozilla had some of the answer.
Additional topics discussed:
- String() function (converts data to a string, numbers gain a length. Dates become a string with the date, time and time zone.)
- .toString method (same as String () function)
- typeof function
- .length method
- .toFixed method (turns a string into a number – number of decimal spaces can be specified. Booleans gain a value of 1 for true and 0 for false. Null values become 0. Strings and arrays gain a value of NaN, which means Not A Number.)
- parseInt function (returns an integer value)
- parseFloat function (returns a decimal value)
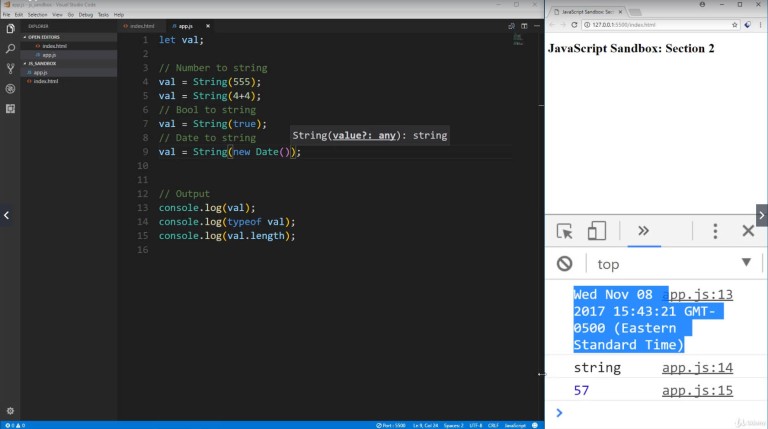
Ok. This post is getting long, so I’m going to break it into 3 parts and cover the remaining topics from JavaScript Fundamentals. Here’s an index of posts about Lambda’s pre-course. Many of the topics covered in the pre-course were discussed in Modern JS. JavaScript starts at post #7.
Nice post, I’ll have to try that “ !” Shortcut that loads an HTML template. I’ve been just typing it out.
LikeLiked by 1 person